通过Windows Azure,你可以使用一个虚拟机来处理计算密集型任务。前面给大家介绍了云平台上创建一个虚拟机和云平台上创建一个服务总线命名空间,本文将给您介绍一下如何创建一个执行计算密集型任务的Java应用程序。
1. 在你的开发机器(并不一定是你所创建的虚拟机)上,为Java下载Windows Azure SDK。
2. 在本小节的末尾创建一个Java控制台应用程序使用的示例代码。在本教程中,我们将使用TSPSolver.java作为java文件的名字。修改your_service_bus_namespace、 your_service_bus_owner、 以及 your_service_bus_key 等占位符,分别使用您的服务总线命名空间、 默认发行人和默认密钥值。
3. 编码后,导出应用程序到一个可运行的Java归档(JAR),并把所需的库到打包已生成的JAR。在本教程中,我们将使用TSPSolver.jar作为生成的JAR名称。
// TSPSolver.java import com.microsoft.windowsazure.services.core.Configuration; import com.microsoft.windowsazure.services.core.ServiceException; import com.microsoft.windowsazure.services.serviceBus.*; import com.microsoft.windowsazure.services.serviceBus.models.*; import java.io.*; import java.text.DateFormat; import java.text.SimpleDateFormat; import java.util.ArrayList; import java.util.Date; import java.util.List; public class TSPSolver { // Value specifying how often to provide an update to the console. private static long loopCheck = 100000000; private static long nTimes = 0, nLoops=0; private static double[][] distances; private static String[] cityNames; private static int[] bestOrder; private static double minDistance; private static ServiceBusContract service; private static void buildDistances(String fileLocation, int numCities) throws Exception{ try{ BufferedReader file = new BufferedReader(new InputStreamReader(new DataInputStream(new FileInputStream(new File(fileLocation))))); double[][] cityLocs = new double[numCities][2]; for (int i = 0; i<numCities; i++){ String[] line = file.readLine().split(“, “); cityNames[i] = line[0]; cityLocs[i][0] = Double.parseDouble(line[1]); cityLocs[i][1] = Double.parseDouble(line[2]); } for (int i = 0; i<numCities; i++){ for (int j = i; j<numCities; j++){ distances[i][j] = Math.hypot(Math.abs(cityLocs[i][0] – cityLocs[j][0]), Math.abs(cityLocs[i][1] – cityLocs[j][1])); distances[j][i] = distances[i][j]; } } } catch (Exception e){ throw e; } } private static void permutation(List<Integer> startCities, double distSoFar, List<Integer> restCities) throws Exception { try { nTimes++; if (nTimes == loopCheck) { nLoops++; nTimes = 0; DateFormat dateFormat = new SimpleDateFormat(“MM/dd/yyyy HH:mm:ss”); Date date = new Date(); System.out.print(“Current time is ” + dateFormat.format(date) + “. “); System.out.println( “Completed ” + nLoops + ” iterations of size of ” + loopCheck + “.”); } if ((restCities.size() == 1) && ((minDistance == -1) || (distSoFar + distances[restCities.get(0)][startCities.get(0)] + distances[restCities.get(0)][startCities.get(startCities.size()-1)] < minDistance))){ startCities.add(restCities.get(0)); newBestDistance(startCities, distSoFar + distances[restCities.get(0)][startCities.get(0)] + distances[restCities.get(0)][startCities.get(startCities.size()-2)]); startCities.remove(startCities.size()-1); } else{ for (int i=0; i<restCities.size(); i++){ startCities.add(restCities.get(0)); restCities.remove(0); permutation(startCities, distSoFar + distances[startCities.get(startCities.size()-1)][startCities.get(startCities.size()-2)],restCities); restCities.add(startCities.get(startCities.size()-1)); startCities.remove(startCities.size()-1); } } } catch (Exception e) { throw e; } } private static void newBestDistance(List<Integer> cities, double distance) throws ServiceException, Exception { try { minDistance = distance; String cityList = “Shortest distance is “+minDistance+”, with route: “; for (int i = 0; i<bestOrder.length; i++){ bestOrder[i] = cities.get(i); cityList += cityNames[bestOrder[i]]; if (i != bestOrder.length -1) cityList += “, “; } System.out.println(cityList); service.sendQueueMessage(“TSPQueue”, new BrokeredMessage(cityList)); } catch (ServiceException se) { throw se; } catch (Exception e) { throw e; } } public static void main(String args[]){ try { Configuration config = ServiceBusConfiguration.configureWithWrapAuthentication( “your_service_bus_namespace”, “your_service_bus_owner”, “your_service_bus_key”); service = ServiceBusService.create(config); int numCities = 10; // Use as the default, if no value is specified at command line. if (args.length != 0) { if (args[0].toLowerCase().compareTo(“createqueue”)==0) { // No processing to occur other than creating the queue. QueueInfo queueInfo = new QueueInfo(“TSPQueue”); service.createQueue(queueInfo); System.out.println(“Queue named TSPQueue was created.”); System.exit(0); } if (args[0].toLowerCase().compareTo(“deletequeue”)==0) { // No processing to occur other than deleting the queue. service.deleteQueue(“TSPQueue”); System.out.println(“Queue named TSPQueue was deleted.”); System.exit(0); } // Neither creating or deleting a queue. // Assume the value passed in is the number of cities to solve. numCities = Integer.valueOf(args[0]); } System.out.println(“Running for ” + numCities + ” cities.”); List<Integer> startCities = new ArrayList<Integer>(); List<Integer> restCities = new ArrayList<Integer>(); startCities.add(0); for(int i = 1; i<numCities; i++) restCities.add(i); distances = new double[numCities][numCities]; cityNames = new String[numCities]; buildDistances(“c:\TSP\cities.txt”, numCities); minDistance = -1; bestOrder = new int[numCities]; permutation(startCities, 0, restCities); System.out.println(“Final solution found!”); service.sendQueueMessage(“TSPQueue”, new BrokeredMessage(“Complete”)); } catch (ServiceException se) { System.out.println(se.getMessage()); se.printStackTrace(); System.exit(-1); } catch (Exception e) { System.out.println(e.getMessage()); e.printStackTrace(); System.exit(-1); } } } |
如何创建一个监视计算密集型任务进展情况的Java应用程序
1. 在你的开发机器上,创建一个Java控制台应用程序,使用的示例代码在本小节的末尾。在本教程中,我们将使用TSPClient.java作为Java文件名。和之前一样,分别使用你的服务总线命名空间、 默认发行人和默认密钥值,修改your_service_bus_namespace、 your_service_bus_owner、 以及 your_service_bus_key 等占位符。
2. 导出应用程序到一个可执行的JAR,并打包所需的库到生成的JAR。在本教程中,我们将使用TSPClient.jar生成的JAR名。
// TSPClient.java import java.util.Date; import java.text.DateFormat; import java.text.SimpleDateFormat; import com.microsoft.windowsazure.services.serviceBus.*; import com.microsoft.windowsazure.services.serviceBus.models.*; import com.microsoft.windowsazure.services.core.*; public class TSPClient { public static void main(String[] args) { try { DateFormat dateFormat = new SimpleDateFormat(“MM/dd/yyyy HH:mm:ss”); Date date = new Date(); System.out.println(“Starting at ” + dateFormat.format(date) + “.”); String namespace = “your_service_bus_namespace”; String issuer = “your_service_bus_owner”; String key = “your_service_bus_key”; Configuration config; config = ServiceBusConfiguration.configureWithWrapAuthentication( namespace, issuer, key); ServiceBusContract service = ServiceBusService.create(config); BrokeredMessage message; int waitMinutes = 3; // Use as the default, if no value is specified at command line. if (args.length != 0) { waitMinutes = Integer.valueOf(args[0]); } String waitString; waitString = (waitMinutes == 1) ? “minute.” : waitMinutes + ” minutes.”; // This queue must have previously been created. service.getQueue(“TSPQueue”); int numRead; String s = null; while (true) { ReceiveQueueMessageResult resultQM = service.receiveQueueMessage(“TSPQueue”); message = resultQM.getValue(); if (null != message && null != message.getMessageId()) { // Display the queue message. byte[] b = new byte[200]; System.out.print(“From queue: “); s = null; numRead = message.getBody().read(b); while (-1 != numRead) { s = new String(b); ss = s.trim(); System.out.print(s); numRead = message.getBody().read(b); } System.out.println(); if (s.compareTo(“Complete”) == 0) { // No more processing to occur. date = new Date(); System.out.println(“Finished at ” + dateFormat.format(date) + “.”); break; } } else { // The queue is empty. System.out.println(“Queue is empty. Sleeping for another ” + waitString); Thread.sleep(60000 * waitMinutes); } } } catch (ServiceException se) { System.out.println(se.getMessage()); se.printStackTrace(); System.exit(-1); } catch (Exception e) { System.out.println(e.getMessage()); e.printStackTrace(); System.exit(-1); } } } |
我们一直都在努力坚持原创.......请不要一声不吭,就悄悄拿走。
我原创,你原创,我们的内容世界才会更加精彩!
【所有原创内容版权均属TechTarget,欢迎大家转发分享。但未经授权,严禁任何媒体(平面媒体、网络媒体、自媒体等)以及微信公众号复制、转载、摘编或以其他方式进行使用。】
微信公众号
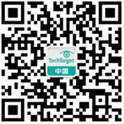
TechTarget
官方微博
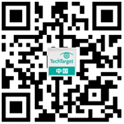
TechTarget中国
相关推荐
-
谷歌云业务CEO描绘谷歌云计划 收购传言四起
行业观察人士猜测,新任谷歌云首席执行官Thomas Kurian将通过大规模收购来获取市场份额,并与竞争对手A […]
-
更新版Oracle Gen 2 Cloud挑战云领导者
通过更新自治数据库技术和其他云服务,甲骨文正在努力增强其Oracle Gen 2 Cloud平台,从而为自己提 […]
-
聚焦新制造,赋能新零售,浪潮云ERP亮相云栖大会
云栖大会在杭州云栖小镇举办,会议主题为“驱动数字中国”,议题涵盖企业智能、智联网、区块链、机器智能等众多前沿创 […]
-
AWS中国(北京)区域正式在中国商用
由光环新网负责运营的AWS中国(北京)区域在中国正式商用,通过这次合作,AWS中国(北京)区域的云服务由光环新网运营和提供,AWS继续向光环新网提供技术支持和专业指导。